Creating a Depth-Aware Boids Animation in React: Flocking with Style (and Parallax!)
Welcome, digital ornithologists and curious React developers! 🪶 Today we're going to take a fun and deep dive into how I built a depth-aware, parallax-speed Boids animation using React and Framer Motion. This is not your average <canvas>
boid soup—this flock is lively, touch-responsive, and fully at home in your next modern React project.
This post is for devs who love animations, crave interactivity, or just want their landing page to whisper, "Yeah, I can code."
What Are Boids Anyway?
Boids are little simulated creatures invented by Craig Reynolds in 1986 to mimic the movement of birds, fish, and all things flocky. The magic comes from three rules:
- Separation: Don't crowd your neighbor.
- Alignment: Fly the way your friends are flying.
- Cohesion: Stay near the group.
The result? Emergent lifelike swarming behavior, beloved by creative coders everywhere.
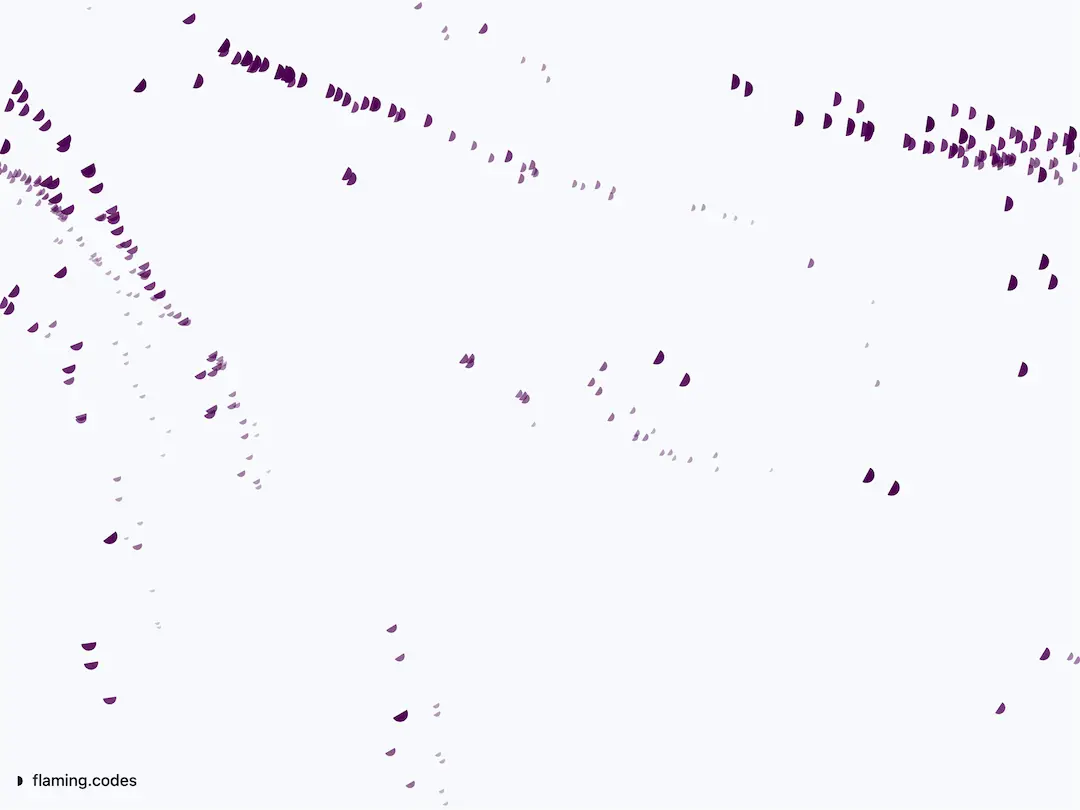
Why React for Boids?
Traditionally, boid simulations live in a canvas or WebGL world. But with today's supercharged UIs and tools like Framer Motion, we can animate DOM nodes efficiently—and get easy React integration, interactivity, and even scroll-based effects as a bonus.
SEO Tip: This approach works great for websites and apps where SEO and accessibility matter—no more hiding your coolest visuals in an inaccessible
<canvas>
!
Component Structure
We wrap our flocking logic inside a single React component, <FlockingAnimation />
. The code is modular and customizable via props, supporting:
- Flock size (auto-calculated or fixed)
- Max speed & force
- Rule weights
- Custom classes for easy styling
Props:
export type Props = {
className?: string;
initialCount?: number;
maxSpeed?: number;
maxForce?: number;
weights?: Partial<Weights>;
};
Boid Behaviors: The Algorithm
Each Boid is a class instance, maintaining its position, velocity, acceleration, and—importantly—its depth for parallax goodness.
The core logic mimics real-life flocking:
flock(boids, weights, maxSpeed, maxForce) {
// 1. Calculate separation, alignment, cohesion vectors
// 2. Weight and sum them
// 3. Apply to acceleration
// 4. Limit acceleration to maxForce
}
Each rule inspects nearby boids within a certain range and calculates a steering vector:
- Separation: Pushes away from boids too close.
- Alignment: Averages velocities to match direction.
- Cohesion: Steers toward the average position.
Depth and Parallax: Adding the Wow
Each boid gets a random depth (between 0 and 1) on creation. Depth does three things:
- Parallax Speed: Farther boids move slower, closer ones faster.
- Scale: Boids in the foreground are bigger.
- Opacity: Deeper boids are faded, nearer ones are bold.
This is handled in the update loop:
const speedFactor = 1 + (1 - this.depth) * PARALLAX_FACTOR;
const dtScalar = dtBase * speedFactor;
When rendered, each boid's scale and opacity are mapped to its depth, so the flock looks genuinely 3D. It's all the immersion of VR, minus the headaches.
Making It Responsive (and Fun!)
- Scroll-Based Fade: Using
useScroll()
anduseTransform()
, the animation fades out as you scroll, so it never overstays its welcome. - Pointer Interaction: Drag with your mouse/finger and you'll spawn a new boid at your cursor. Because who doesn't want to feel like the Flock Overlord?
- Randomized Flock Distribution: On mount, groups of boids spawn from random centers, spreading naturally.
Example of boid spawning:
for (let j = 0; j < groupSize; j++) {
const ang = Math.random() * Math.PI * 2;
const rad = Math.random() * SPAWN_RADIUS;
boidsRef.current.push(
new Boid(center.x + Math.cos(ang) * rad, center.y + Math.sin(ang) * rad)
);
}
Optimizations and Dev Tips
- Visibility-Aware Rendering: We use an Intersection Observer to pause updates when the component isn't visible, saving CPU cycles and battery life for your mobile users (or your laptop's fans).
- Efficient State Updates: We only bump React state when needed, keeping renders to a minimum.
- Motion Values: Using Framer Motion's
motionValue
and animated<motion.div>
s, we get buttery-smooth transforms in the DOM.
Full Source Code
Find the full, ready-to-plug-in link below! (Just bring your own Framer Motion and Tailwind CSS setup.)
Conclusion
Building flocking boids in React might sound like an oddball choice, but with modern animation libraries, you get interactivity, performance, and that precious developer experience—all.